Any developer who wrote any JavaScript code must have used the console.log() method at some point to log informational messages or for print debugging. However, while that method is quite useful, it is not the only one in our arsenal. So let’s look at some useful methods that are not very commonly known to most developers.
time() / timeLog() / timeEnd()
If you have some long-running tasks and want to get some insights into how long a task takes, these methods are handy. You can start a timer by calling:
console.time();
and end the timer by calling
console.timeEnd();
You can also pass a label to these methods to make the logs more readable. Otherwise, the times are logged under the “default” label.
timeLog() method can be used anywhere between time and timeEnd to log the timer’s current value.
The example below uses all the features discussed above:
console.time(); for (let i = 0; i < 10000000; i++) { if (i === 5000000) { console.timeLog(); } } console.timeEnd();
It loops 10 million times and logs the time halfway through (console.timeLog()) and at the end (console.timeEnd())
and the output looks like this:

table()
This neat little feature can be useful when displaying tabular data. In this example, we are going to get the first ten results from people endpoint on Star Wars API and display them in a table:
fetch ("https://swapi.py4e.com/api/people/") .then(data => data.text()) .then(response => { console.table(JSON.parse(response).results); });
Let’s check out the results:

And all it takes is five lines of code to produce this!
warn() / error()
console.log() is fine for logging informational statements, but to make the warning and errors more readable, you can use warn() and error() methods. When checking the console, they stand out among all the other log lines.
For example, the following code:
for (let i = 1; i <= 10; i++) { console.log(i); if (i === 5) { console.warn("warning: half-way through"); } } console.error("error: all gone now");
produces this output:

debug()
I chose to cover debug in a separate section even though it works very similarly to log(), warn() and error() methods. By default, the log level in browsers is not set to display debug messages.

So if we run the previous example with log() call replaced by debug() call, the output looks very simplified:
for (let i = 1; i <= 10; i++) { console.debug(i); if (i === 5) { console.warn("warning: half-way through"); } } console.error("error: all gone now");
Output with debugging:

If we want to see all the statements, we need to explicitly set the logging level to verbose and get the same results as before.

group() / groupEnd() / groupCollapsed()
group() and groupEnd() let us create indented log entries within a group label.
For example, the following code
console.group("Group 1"); console.log("entry 1"); console.log("entry 2"); console.log("entry 3"); console.groupEnd("Group 1"); console.group("Group 2"); console.log("entry 1"); console.log("entry 2"); console.log("entry 3"); console.groupEnd("Group 2");
produces this result:
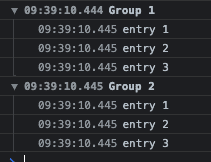
We can also create log entries in a collapsed state so that they can only be viewed when we explicitly expand the log group:
console.group("Group 1"); console.log("entry 1"); console.log("entry 2"); console.log("entry 3"); console.groupCollapsed("Hidden stuff until you expand"); console.log("Hidden entry 1"); console.log("Hidden entry 2"); console.groupEnd("Group 1");
By default, the output looks like this:

To see the contents of the collapsed group, we need to expand it explicitly:

Conclusion
This post looked into useful Console API methods that are not commonly known. To ensure compatibility, we didn’t look into non-standard methods such as timeStamp() and profile(). Instead, I’d recommend visiting the resources below and looking into all the methods. Some of them are not covered here but might be valuable to you.